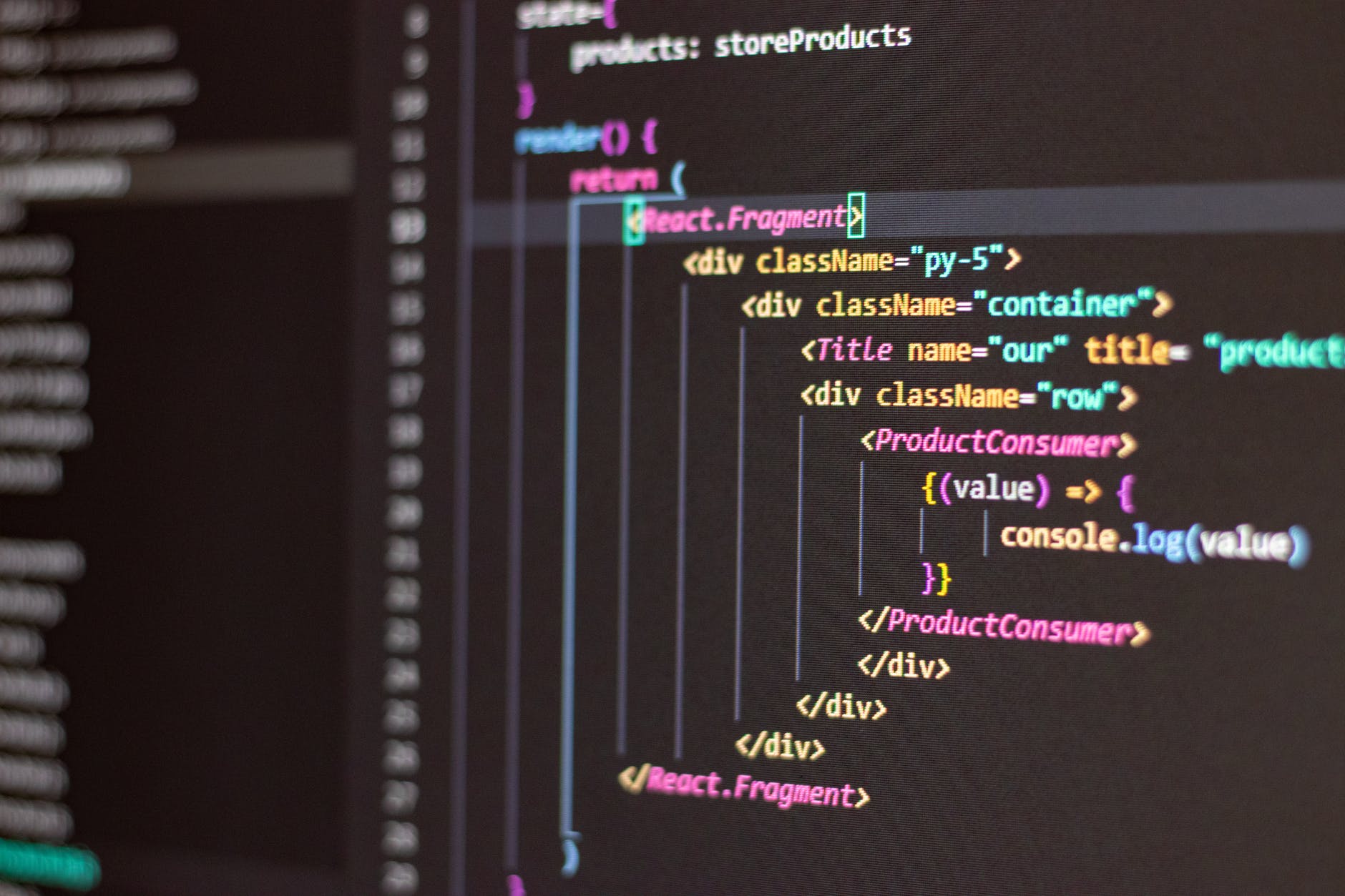
As you all know, when working with code for Microsoft Dataverse we have two options late-bound and early-bound. I have seen many people advocating one option over another and explaining why one is better than the other. For me, it’s just a personal choice, if you prefer typed classes go for early bound else go for the late-bound approach. Add some additional details below to help you to select a programming option.
Early Bound
Early-bound programming requires that you first generate a set of classes based on the table and column definitions (entity and attribute schema) for a specific environment using the code generation tool. Once generated you can enjoy better IntelliSense and compile-time checking of all types so that no implicit casts occur. Below is the sample early bind code from Microsoft Docs which creates an account record.
var account = new Account();
// set attribute values
// string primary name
account.Name = "Contoso";
// Boolean (Two option)
account.CreditOnHold = false;
// DateTime
account.LastOnHoldTime = new DateTime(2017, 1, 1);
// Double
account.Address1_Latitude = 47.642311;
account.Address1_Longitude = -122.136841;
// Int
account.NumberOfEmployees = 500;
// Money
account.Revenue = new Money(new decimal(5000000.00));
// Picklist (Option set)
account.AccountCategoryCode = new OptionSetValue(1); //Preferred customer
//Create the account
Guid accountid = svc.Create(account);
Late Bound
Here you don’t need to generate the classes, you directly start wrting your logic, However, you need to refer to tables and columns (entities and attributes) using their LogicalNames in the Entity class. You don’t get compile-time validation on names of entities, attributes, and relationships. Types are checked only when the object is created. Below is the sample late bind code from Microsoft Docs which creates an account record.
//Use Entity class specifying the entity logical name
var account = new Entity("account");
// set attribute values
// string primary name
account["name"] = "Contoso";
// Boolean (Two option)
account["creditonhold"] = false;
// DateTime
account["lastonholdtime"] = new DateTime(2017, 1, 1);
// Double
account["address1_latitude"] = 47.642311;
account["address1_longitude"] = -122.136841;
// Int
account["numberofemployees"] = 500;
// Money
account["revenue"] = new Money(new decimal(5000000.00));
// Picklist (Option set)
account["accountcategorycode"] = new OptionSetValue(1); //Preferred customer
//Create the account
Guid accountid = svc.Create(account);
Now let’s do a comparison on both.
Early-bound | Late-bound |
---|---|
You can verify entity, attribute, and relationship names at compile time | No compile-time verification of entity, attribute, and relationship names |
You must generate entity classes | You don’t need to generate entity classes |
Better IntelliSense support | Less IntelliSense support |
Less readable code | More readable code |
Slightly less performant | Slightly more performant |
Generated classes are quite large | Generated classes are small |
Hope this helps.